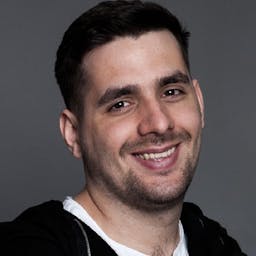
Janek Kruczkowski
Unraveling Named and Default Exports in JavaScript: A Comprehensive Guide
Introduction
In the world of JavaScript, developers often face the challenge of managing and organizing their code. To help with this, ES6 introduced the module system, which has become an essential tool for maintaining clean and scalable codebases. In this comprehensive guide, we will explore the core differences between named and default exports in JavaScript and provide you with practical examples and insights to use them effectively in your projects.
Understanding exports in JavaScript
JavaScript modules are a way to organize and share code between different parts of your application. There are two types of exports in JavaScript:
- Named exports
- Default exports
Let's dive deeper into each one of them.
Named exports
Named exports allow you to export multiple variables, functions, or objects from a module. Each exported entity has a unique name that can be used to import it in other parts of your application.
Declaring named exports:
// utils.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
Importing named exports:
// app.js
import { add, subtract } from './utils.js';
console.log(add(3, 4)); // 7
console.log(subtract(7, 4)); // 3
Default exports
Default exports allow you to export a single entity from a module, which becomes the default export for that module. This can be a function, object, or variable.
Declaring default exports:
// math.js
const multiply = (a, b) => a * b;
export default multiply;
Importing default exports:
// app.js
import multiply from './math.js';
console.log(multiply(3, 4)); // 12
Differences between named and default exports
There are several key differences between named and default exports:
-
Syntax: Named exports require the
export
keyword, followed by the entity being exported, while default exports use theexport default
keyword. -
Importing: Named exports must be imported using the exact name enclosed in curly braces, while default exports can be imported using any name without curly braces.
-
Use cases: Named exports are ideal for exporting multiple entities from a module, while default exports are suitable when a module has a primary entity to export
Combining named and default exports
In some cases, you might want to use both named and default exports in the same module. This can be achieved by exporting multiple named entities alongside a default export.
Example:
// calculator.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
const calculator = {
add,
subtract,
};
export default calculator;
Importing combined exports:
// app.js
import calculator, { add, subtract } from './calculator.js';
console.log(calculator.add(3, 4)); // 7
console.log(add(3, 4)); // 7
console.log(subtract(7, 4)); // 3
Best practices for using named and default exports
- Use named exports for utility functions or objects that can be used independently.
- Use default exports for the primary entity of a module, such as a class or a library.
- When using both named and default exports, ensure that the default export is the most important or frequently used entity.
Common pitfalls and how to avoid them
-
Inconsistent naming: When importing named exports, it's important to use the exact name to avoid errors. Ensure that you and your team maintain consistent naming conventions.
-
Misusing default exports: Avoid using default exports for utility functions or objects that are not the primary focus of a module.
Real-world scenarios
Example 1: Using named exports
Imagine you are building a utility library for working with arrays. Named exports are ideal for this scenario since each function can be used independently.
// arrayUtils.js
export const sum = (arr) => arr.reduce((acc, curr) => acc + curr, 0);
export const average = (arr) => sum(arr) / arr.length;
Example 2: Using default exports
Suppose you are building a library for managing state in a JavaScript application. In this case, the primary entity would be the state management class, which would be a good candidate for a default export.
// stateManager.js
class StateManager {
constructor(initialState) {
this.state = initialState;
}
// Other methods for managing state
}
export default StateManager;
How to migrate from CommonJS to ES6 modules
- Replace
module.exports
withexport default
for single exports. - Replace
exports.name = value
withexport const name = value
for named exports. - Replace
require()
withimport
for importing modules.
Browser support for ES6 modules
Modern browsers like Chrome, Firefox, Safari, and Edge support ES6 modules by default. However, for older browsers like Internet Explorer, you might need to use a bundler like Webpack or Rollup to convert your code into a format that older browsers can understand.
Tools and resources for working with JavaScript modules
- Webpack - A popular bundler and build tool for JavaScript applications.
- Rollup - A module bundler that focuses on simplicity and performance.
- Babel - A JavaScript compiler that allows you to use the latest features in older browsers.
Summary
In this article, we have explored the key differences between named and default exports in JavaScript and provided examples and best practices for using them effectively in your projects. By understanding these concepts, you can write more modular, maintainable, and scalable code.
Conclusion
Named and default exports are essential tools for organizing and sharing
code in JavaScript applications. By understanding their differences and use cases, you can better decide which export type to use in your projects. Remember to follow best practices and be mindful of common pitfalls to maintain clean and scalable codebases.
FAQs
- What is the main difference between named and default exports?
The main difference is that named exports allow you to export multiple entities from a module, while default exports allow you to export a single entity.
- Can I use both named and default exports in the same module?
Yes, you can use both named and default exports in the same module. However, it's important to ensure that the default export is the most important or frequently used entity.
- What is the syntax for importing named and default exports?
Named exports are imported using the exact name enclosed in curly braces, e.g., import { name } from 'module';
. Default exports can be imported using any name without curly braces, e.g., import name from 'module';
.
- Do I need a bundler like Webpack or Rollup for using JavaScript modules?
Modern browsers support ES6 modules natively. However, for older browsers or complex applications, you might need a bundler like Webpack or Rollup to handle module bundling and optimization.
- How do I migrate from CommonJS to ES6 modules?
To migrate from CommonJS to ES6 modules, replace module.exports
with export default
, replace exports.name = value
with export const name = value
, and replace require()
with import
for importing modules.