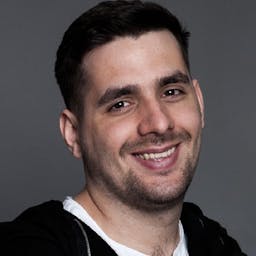
Janek Kruczkowski
Differences between TypeScript Interfaces vs. Types
In this article, we'll explore the differences between TypeScript interfaces and types. We'll start with a brief introduction to TypeScript and then dive into interfaces and types, their similarities, and differences. We'll also discuss when to use each of them and provide some real-world use cases and code examples. Let's get started!
Understanding TypeScript
What is TypeScript?
TypeScript is a statically typed superset of JavaScript developed by Microsoft. It adds optional type annotations and other features to the language, making it more robust and easier to maintain. TypeScript code is transpiled to JavaScript, allowing it to run in any JavaScript environment.
Why TypeScript?
TypeScript offers several benefits over vanilla JavaScript, such as:
- Improved code quality and maintainability
- Easier debugging
- Better tooling and developer experience
- Enhanced scalability for large projects
TypeScript Interfaces
Definition and Usage
An interface in TypeScript is a way to define a contract for the shape of an object. It specifies the properties and methods that an object should have, without providing any implementation details. Interfaces are used to enforce consistency and improve code readability.
Interface Examples
interface Person {
name: string;
age: number;
greet(): void;
}
const john: Person = {
name: "John Doe",
age: 30,
greet() {
console.log(`Hello, my name is ${this.name}!`);
},
};
john.greet();
// Output: Hello, my name is John Doe!
TypeScript Types
Definition and Usage
In TypeScript, the type
keyword is used to define custom types, which can be composed of primitive types, union types, intersection types, and more. Types can represent a wide range of constructs and can be used to enforce type safety and code consistency.
Type Examples
type Vehicle = {
make: string;
model: string;
year: number;
};
const car: Vehicle = {
make: "Toyota",
model: "Camry",
year: 2020,
};
Interfaces vs. Types
Similarities
Both interfaces and types can be used to describe the shape of objects and enforce type safety in TypeScript. They can be used in a similar way when defining the structure of an object.
Differences
-
Declaration Merging: Interfaces support declaration merging, while types do not. This means that you can declare multiple interfaces with the same name, and they will be merged into a single definition. This is not possible with types.
-
Extensibility: Interfaces can be extended using the
extends
keyword, while types can be combined using intersection types. -
Implementation: Classes can implement interfaces, ensuring that they adhere to the defined contract. This is not possible with types.
-
Syntax: Interfaces use a slightly different syntax compared to types. Interfaces use the
interface
keyword, while types use thetype
keyword.
When to Use Interfaces
You should consider using interfaces when:
- Defining contracts for classes and objects.
- You need declaration merging or extensibility.
- You want to improve code readability by explicitly defining the structure of an object.
When to Use Types
You should consider using types when:
- Defining complex or reusable types, such as union or intersection types.
- You require more flexibility in defining shapes.
- You want to create aliases for existing types.
Combining Interfaces and Types
Interfaces and types can be combined to create more powerful and flexible constructs in TypeScript. For example, you can use interfaces to define the shape of an object and types to define a union type of different interfaces.
interface Car {
type: "car";
wheels: number;
}
interface Bicycle {
type: "bicycle";
wheels: number;
}
type Vehicle = Car | Bicycle;
const myVehicle: Vehicle = {
type: "car",
wheels: 4,
};
Real-World Use Cases
Interface Use Case
Interfaces are useful for creating contracts for classes in a large project. For example, if you have a library for handling user authentication, you can define an interface for the user class:
interface IUser {
id: string;
username: string;
password: string;
authenticate(password: string): boolean;
}
class User implements IUser {
// implementation details...
}
Type Use Case
Types are great for creating complex types that represent different possibilities. For example, in a graphics library, you could define a type to represent different shapes:
type Point = [number, number];
type Circle = {
kind: "circle";
center: Point;
radius: number;
};
type Rectangle = {
kind: "rectangle";
topLeft: Point;
bottomRight: Point;
};
type Shape = Circle | Rectangle;
Conclusion
In summary, TypeScript interfaces and types are powerful tools for enforcing type safety, consistency, and readability in your code. While they have similarities, they also have key differences that make each more suitable for specific use cases. By understanding when to use each and how to combine them effectively, you can create cleaner and more maintainable TypeScript projects.
FAQs
-
Can I use both interfaces and types in my TypeScript project? Yes, you can use both interfaces and types in your project based on your requirements and specific use cases.
-
Can interfaces and types be used interchangeably? While they have some similarities, interfaces and types have different features and use cases. Choose the appropriate one based on your needs.
-
How do I choose between using an interface or a type? Consider using interfaces for defining contracts and improving readability, and types for more complex and flexible type definitions.
-
Can interfaces extend types? No, interfaces can only extend other interfaces. However, you can use intersection types to combine types with interfaces.
-
What happens when I have conflicting interface declarations with the same name? TypeScript will merge the declarations into a single interface, combining the properties and methods of both interfaces.