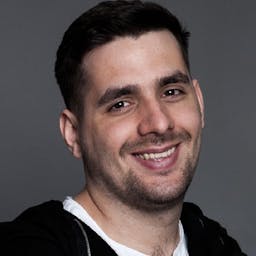
Janek Kruczkowski
A Comprehensive Guide for Beginners Diving into React.js Development
Introduction to React.js
What is React.js?
React.js, or simply React, is an open-source JavaScript library developed by Facebook for building user interfaces (UIs) in web applications. React makes it easier to create reusable UI components, manage the application state, and handle user interactions.
Why choose React.js?
React has become one of the most popular web development frameworks for several reasons:
- Reusable components: React allows you to build modular, reusable UI components that simplify development and improve maintainability.
- Virtual DOM: React uses a virtual DOM to efficiently update the UI, resulting in better performance and a smoother user experience.
- Strong community and ecosystem: React has a large, active community, which means you'll find plenty of resources, tutorials, and third-party libraries to help you along the way.
Setting Up Your Development Environment
Node.js and npm
Before diving into React development, you'll need to set up your development environment. First, install Node.js and npm (Node Package Manager), which comes bundled with Node.js. You'll use npm to install and manage dependencies for your React projects.
Create React App
Next, install the Create React App CLI tool, which is the official way to start a new React project:
npm install -g create-react-app
With Create React App, you can easily generate a new React project with a sensible default configuration:
create-react-app my-react-app
cd my-react-app
npm start
This will create a new directory called my-react-app
, install the necessary dependencies, and start a development server. Open your browser to http://localhost:3000/
, and you should see the default React app running.
Understanding React.js Fundamentals
Components
In React, a component is a self-contained piece of the UI that can be easily reused and combined with other components to build more complex UIs. Components can be created as JavaScript functions or classes.
Here's an example of a simple functional component:
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
JSX
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code within your
JavaScript code. React components often use JSX to define their UI structure and appearance. Here's an example of JSX code:
const element = <h1>Hello, world!</h1>;
State and Props
In React, components can have their own internal state and receive external data through props. State represents the component's internal data, which can change over time, while props represent the data passed from a parent component.
Here's an example of a simple class component with state and props:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return (
<div>
<h1>{this.props.title}</h1>
<p>Count: {this.state.count}</p>
</div>
);
}
}
Event Handling
React components can handle user events, such as clicks, keyboard input, and form submissions, using event handlers. Event handlers are functions that are called when an event occurs. Here's an example of a button with an onClick event handler:
class Button extends React.Component {
handleClick() {
console.log("Button clicked");
}
render() {
return (
<button onClick={this.handleClick}>
Click me
</button>
);
}
}
Working with Components
Functional Components
Functional components are simpler and more concise than class components. They're just JavaScript functions that take props as input and return a React element. Here's an example of a functional component:
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
Class Components
Class components are more complex than functional components, but they offer additional features, such as state and lifecycle methods. Here's an example of a class component:
class Timer extends React.Component {
constructor(props) {
super(props);
this.state = { time: 0 };
}
render() {
return <h1>Time: {this.state.time} seconds</h1>;
}
}
Lifecycle Methods
Class components have several lifecycle methods that you can use to manage the component's state and perform side effects, such as fetching data or updating the DOM. Some common lifecycle methods include componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
React.js Best Practices
Code Organization
Organize your React code into a clear and consistent directory structure, separating components, styles, and utility functions into their own files and directories. This makes your code more maintainable and easier to understand.
Performance Optimizations
Optimize your React application's performance by using techniques such as:
- Lazy loading components
- Debouncing or throttling event handlers
- Using the
React.memo
function to memoize functional components - Using the
shouldComponentUpdate
lifecycle method in class components
Accessibility
Make your React application accessible to all users by following accessibility best practices, such as:
- Adding meaningful alt text to images
- Using semantic HTML elements
- Providing keyboard navigation
- Implementing ARIA attributes
Building a Simple React.js Application
Planning Your App
Before diving into code, plan your application by sketching out the UI and listing the required components and their functionality.
Setting Up the Project
Create a new React project using Create React App and install any necessary dependencies using npm.
Developing Components
Start by developing the individual components of your application, such as buttons, forms, and navigation elements. Focus on making your components reusable and modular.
Implementing Interactivity
Once your components are in place, add interactivity to your application by implementing event handlers, managing state, and handling user input.
Connecting Components
Now that your components are interactive, connect them together to form a complete application. Use props to pass data between components and manage your application's state in a top-level component or using a state management library like Redux.
Conclusion
In this comprehensive guide, we've covered the basics of React.js development for beginners, including setting up your development environment, understanding React.js fundamentals, working with components, following best practices, and building a simple React.js application. With these concepts in hand, you're ready to dive deeper into React.js development and build amazing web applications.
Frequently Asked Questions
1. What are the main differences between functional and class components in React.js?
Functional components are simpler, stateless, and more concise, while class components offer additional features like state and lifecycle methods. With the introduction of hooks, functional components can now also have state and side effects.
2. What is the Virtual DOM, and how does it benefit React.js applications?
The Virtual DOM is an in-memory representation of the actual DOM that React.js uses to efficiently update the UI. By using the Virtual DOM, React can minimize the number of costly DOM manipulations, resulting in better performance and a smoother user experience.
3. Can I use React.js with other JavaScript libraries and frameworks?
Yes, React.js can be used alongside other JavaScript libraries and frameworks. However, it's essential to ensure that there are no conflicts between React's DOM updates and other libraries that manipulate the DOM.
4. How do I manage the global state of my React.js application?
While React components can manage their own local state, you might want to use a state management library like Redux or MobX for managing global application state. These libraries provide a centralized store for your application's state and help manage state updates more predictably.
5. Can I use React.js for mobile app development?
Yes, you can use React Native, a sister project of React.js, to build native mobile applications for iOS and Android platforms. React Native uses the same component-based architecture and allows you to share much of your React.js knowledge and code across web and mobile applications.