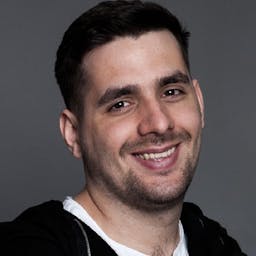
Janek Kruczkowski
React Differences Between useEffect vs useLayoutEffect
React hooks are an essential part of creating functional components in React. They are used to add state, side effects, and other custom logic to your application. Two of the most popular hooks are useEffect
and useLayoutEffect
. Although they might seem similar, they are quite different in how they behave and operate.
In this article, we'll explore these two hooks' differences and when to use them appropriately.
What are useEffect and useLayoutEffect?
useEffect
is a hook that allows you to run side-effects after the component has rendered. Side-effects may include fetching data from a remote server, registering an event listener or setting up a timer. You can also use useEffect
to keep track of changes to specific variables in your state.
useLayoutEffect
is another hook that allows you to run side-effects after the component has rendered, but before the browser paints the screen. That means that any side-effect you run with useLayoutEffect
will block the browser's rendering process, which might impact the performance.
Differences between useEffect and useLayoutEffect
The main difference between useEffect
and useLayoutEffect
is the timing of when their effects are executed. As we mentioned earlier, useEffect
runs the side effects after the component has rendered and painted on the screen. In contrast, useLayoutEffect
runs the side-effects before the app gets painted on the screen, just before the user sees the output.
Another difference is how they handle render-blocking issues caused by their effects. useEffect
handles these issues asynchronously, meaning that the function returned from useEffect runs after the rendering cycle finishes. useLayoutEffect
, on the other hand, handles these issues synchronously, meaning that it runs before the painting process but can cause blocking if the effect takes a long time to execute.
When to use useEffect
If you don't have to manipulate the DOM or perform heavy calculations, useEffect
is the hook you should use. This is because the effect you run with useEffect
is asynchronous, meaning it won't block the rendering process, resulting in better performance. useEffect should be used when you want to perform cleanup tasks such as removing event listeners or when you want to update your state.
import React, { useState, useEffect } from "react";
export default function App() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log("Component mounted");
return () => console.log("Component unmounted");
}, []);
return (
<>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</>
);
}
The above example logs the message "Component mounted" when the component has rendered and logs "Component unmounted" when the component is being removed from the DOM.
When to use useLayoutEffect
useLayoutEffect
should be used when you want to perform any action that is tightly coupled with the DOM elements or impacts the UI. This is because useLayoutEffect
runs just before the app gets painted on the screen, so you can use it to read and manipulate the layout of the elements in the DOM.
import React, { useLayoutEffect, useState } from "react";
export default function App() {
const [count, setCount] = useState(0);
const [color, setColor] = useState("white");
useLayoutEffect(() => {
if (count % 2 === 0) {
setColor("pink");
} else {
setColor("green");
}
}, [count]);
return (
<>
<p style={{ backgroundColor: color }}>
You clicked {count} times and the background color is {color}
</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</>
);
}
In this example, the color of the paragraph's background changes based on the count's value. This is achieved by the useLayoutEffect
effect changing the color state variable. Since we want to run the effect only when the count state changes, we pass the count
variable as a dependency to the effect.
Conclusion
In summary, useEffect
and useLayoutEffect
are two powerful hooks that you can use to manage functionality in your React components. Knowing the differences between these hooks will allow you to choose the hook that better suits your needs. Remember that, as always, the performance impact is something to consider when using these hooks.
FAQs
- What is the difference between
useEffect
anduseLayoutEffect
?useEffect
runs the side effects after rendering, whileuseLayoutEffect
runs side effects before rendering.
- How do I choose the right hook between
useEffect
anduseLayoutEffect
?- If your effect doesn't manipulate the UI, use
useEffect
. If it does, useuseLayoutEffect
.
- If your effect doesn't manipulate the UI, use
- Can I use
useEffect
anduseLayoutEffect
together in the same component?- Yes, you can. However, you should be careful with those hooks and use them appropriately.
- How many times does
useLayoutEffect
run?useLayoutEffect
runs after every render, always before the browser paints the screen.
- How do I stop
useEffect
from running every time my component renders?- You can pass a dependency array to
useEffect
to tell it when to run. If it's empty, it will only run when the component mounts and unmounts.
- You can pass a dependency array to