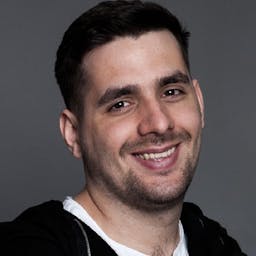
Janek Kruczkowski
Integrating React with Chat GPT for Powerful Conversational UIs
Introduction
In today's world, the success of web applications is largely dependent on their ability to provide engaging and intuitive user interfaces. One such interface that is gaining popularity is the conversational UI. With the help of chatbots powered by natural language understanding (NLU) and natural language processing (NLP) techniques, conversational UIs enable users to interact with web applications in a more human-like way. In this article, we will explore how to integrate React with Chat GPT for creating powerful conversational UIs.
What is Chat GPT?
Chat GPT (Generative Pretrained Transformer) is an NLP model developed by OpenAI. It is a general-purpose language model that can perform a wide variety of NLP tasks such as text classification, sentiment analysis, and language modeling. Chat GPT is best known for its ability to generate human-like text, making it ideal for chatbots and conversational AI applications.
Why Use React for Conversational UIs?
React is a popular JavaScript library for building user interfaces. It is known for its declarative and component-based programming paradigms that make it easy to build reusable UI components. React is also highly flexible, which makes it ideal for integrating with other libraries and frameworks like Chat GPT.
Integrating Chat GPT with React
To integrate Chat GPT with React, we first need to install the required dependencies. We can use the following command to install the @openai/api
package:
npm install @openai/api
After installing the package, we can import it in our React component like this:
import openai from '@openai/api';
Next, we need to create an instance of the OpenAI API client by providing our API key. We can do this by calling the create
method of the openai
object:
const openai = require('openai');
const api_key = 'my_api_key';
const openai = new openai(api_key);
Once we have created an instance of the OpenAI API client, we can use it to generate text using Chat GPT. For example, to generate a response to a user's input, we can call the openai.complete
method:
const prompt = 'Hello, how can I help you today?';
const completions = await openai.complete({
engine: 'text-davinci-002',
prompt: prompt,
maxTokens: 50,
n: 1,
stop: ['\n', 'User:'],
});
const message = completions.choices[0].text.trim();
In the above code, we provide a prompt to the complete
method and specify the Chat GPT engine to use. We also limit the maximum number of tokens in the generated text to 50 and specify that we only want one completion. Finally, we define the stop sequence to indicate when the generated text should stop.
Creating a Conversational UI Component with React and Chat GPT
With the integration of Chat GPT and React in place, we can create powerful conversational UI components for our web applications. To create a basic chatbot interface, we can create a new React component and use the useState
hook to manage the user's input and the bot's responses:
const [messages, setMessages] = useState([]);
const [inputMessage, setInputMessage] = useState('');
const handleInputSubmit = async (e) => {
e.preventDefault();
const message = inputMessage.trim();
if (!message) {
return;
}
setMessages([...messages, { text: message, isUser: true }]);
setInputMessage('');
const completions = await openai.complete({
engine: 'text-davinci-002',
prompt: `User: ${message}\nBot:`,
maxTokens: 50,
n: 1,
stop: ['\n', 'User:'],
});
const botMessage = completions.choices[0].text.trim();
setMessages([...messages, { text: botMessage, isUser: false }]);
};
In the above code, we define the state variables for the user's input and the bot's responses. We also define a handler function for submitting the user's input. Inside the handler function, we first check if the user's input is not empty. Then, we update the messages
state array with the user's input. Next, we call the complete
method of the OpenAI API client to generate a response to the user's input. Finally, we update the messages
state array with the bot's response.
To render the chatbot UI, we can use the following JSX code:
<div className="messages-container">
{messages.map((message, i) => (
<div key={i} className={`message ${message.isUser ? 'user' : 'bot'}`}>
{message.text}
</div>
))}
</div>
<form className="input-form" onSubmit={handleInputSubmit}>
<input
type="text"
placeholder="Type your message..."
value={inputMessage}
onChange={(e) => setInputMessage(e.target.value)}
/>
<button type="submit">Send</button>
</form>
The above code creates a container for displaying the chat messages and a form for accepting user input. We map through the messages
state array and render each message as a separate div
element with a CSS class based on whether it is a user or bot message. We also attach an onChange
event listener to the input field to update the inputMessage
state variable and call the handleInputSubmit
function when the form is submitted.
Conclusion
In this article, we learned how to integrate React with Chat GPT to create powerful and engaging conversational UIs for our web applications. By combining the declarative and component-based programming paradigms of React with the natural language understanding and natural language processing capabilities of Chat GPT, we can create chatbots that are both intuitive and intelligent.
FAQs
-
What is Chat GPT? Chat GPT is an NLP model developed by OpenAI that is best known for its ability to generate human-like text, making it ideal for chatbots and conversational AI applications.
-
Why use React for conversational UIs? React is a popular JavaScript library for building user interfaces that is highly flexible and can be easily integrated with other libraries and frameworks like Chat GPT.
-
How do I integrate Chat GPT with React? To integrate Chat GPT with React, you first need to install the
@openai/api
package and create an instance of the OpenAI API client. You can then use thecomplete
method of the OpenAI API client to generate text using Chat GPT. -
How do I create a conversational UI component with React and Chat GPT? To create a conversational UI component with React and Chat GPT, you can create a new React component and use the
useState
hook to manage the user's input and the bot's responses. You can then use thecomplete
method of the OpenAI API client to generate a response to the user's input and update the state variables accordingly. -
What are some best practices for creating conversational UIs? Some best practices for creating conversational UIs include designing a natural and intuitive conversation flow, providing clear instructions and feedback to the user, and using a consistent tone and style throughout the conversation.