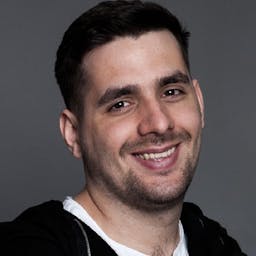
Janek Kruczkowski
Mastering React Strict Mode for Smoother React 18 Upgrades
Outline
- Introduction to React 18 and concurrent rendering
- What is React Strict Mode?
- Importance of Strict Mode in React 18 upgrades
- How to enable React Strict Mode
- Understanding double-invocation in Strict Mode
- How Strict Mode affects useEffect
- Using useRef to manage initial actions in Strict Mode
- Benefits of using Strict Mode in development
- React.StrictMode vs. React.unstable_ConcurrentMode
- Common issues detected by Strict Mode
- Tips for a smoother transition to React 18
- React 18 and backward compatibility
- Gradual adoption of React 18 features
- Exploring other React 18 features
- Conclusion
Introduction to React 18 and concurrent rendering
React 18 introduces a game-changing feature called concurrent rendering. It allows React to prepare multiple versions of your user interface simultaneously, resulting in fluid user experiences even during large rendering tasks. Concurrent rendering is a breaking change, but React 18 aims to provide a gradual adoption path for existing users. One of the tools to help with this transition is React Strict Mode.
What is React Strict Mode?
React Strict Mode is a feature that helps developers identify potential problems in their React applications during development. It doesn't affect production behavior, but during development, it logs extra warnings and double-invokes functions that are expected to be idempotent. This helps prevent common types of mistakes.
Importance of Strict Mode in React 18 upgrades
Strict Mode is an essential tool for developers who are upgrading to React 18 and gradually adopting concurrent features. It highlights concurrency-related bugs during development, helping you ensure that your application works smoothly with the new rendering model.
How to enable React Strict Mode
Enabling Strict Mode is simple. Wrap your application or specific components in <React.StrictMode>
:
import React from 'react';
import { createRoot } from "react-dom/client";
import App from './App';
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
Understanding double-invocation in Strict Mode
When using Strict Mode with the development build, components will be mounted twice. This behavior is intended to help you identify issues caused by non-idempotent functions and side effects.
How Strict Mode affects useEffect
In Strict Mode, useEffect
with an empty dependency array will be called twice, as components are mounted twice. This can lead to unexpected behavior if the effect performs an action that should only happen once.
Using useRef to manage initial actions in Strict Mode
To ensure that an action is performed only initially using useEffect
, you can set a flag using the useRef
hook:
import React, { useEffect, useRef } from 'react';
function ExampleComponent() {
const isInitialMount = useRef(true);
useEffect(() => {
if (isInitialMount.current) {
// Perform your initial action here
isInitialMount.current = false;
}
}, []);
return <div>...</div>;
}
This approach ensures that the desired effect will only run once, even when Strict Mode is enabled
Benefits of using Strict Mode in development
Using Strict Mode during development has several benefits:
- Early detection of potential issues: Strict Mode helps you identify problems related to concurrency and other common mistakes before they cause issues in production.
- Smoother React 18 transition: By addressing issues detected by Strict Mode, you can transition to React 18 with greater confidence.
- Improved application stability: As you fix potential issues surfaced by Strict Mode, your application becomes more stable and resilient.
- Better development practices: Strict Mode encourages you to adopt better development practices, such as idempotent functions and proper management of side effects.
React.StrictMode vs. React.unstable_ConcurrentMode
Before React 18, there was an experimental feature called React.unstable_ConcurrentMode
. This feature was similar to Strict Mode but specifically targeted the then-experimental concurrent rendering features. In React 18, concurrent rendering is stable and fully supported. As a result, React.unstable_ConcurrentMode
has been replaced by React.StrictMode
, which now helps with the transition to React 18's concurrency features.
Common issues detected by Strict Mode
Strict Mode can help identify several types of issues, including:
- Non-idempotent functions or side effects
- Incorrect usage of React hooks
- Deprecated lifecycle methods
- Legacy context API usage
- Mutable external state
By addressing these issues, you can improve the overall quality and maintainability of your React application.
Tips for a smoother transition to React 18
- Enable Strict Mode: Wrap your application or specific components in
<React.StrictMode>
to identify potential issues during development. - Update dependencies: Ensure that your dependencies are compatible with React 18.
- Gradually adopt concurrent features: Take advantage of React 18's gradual adoption path to ensure a smooth transition.
- Refactor code: Address issues detected by Strict Mode and refactor your code to follow best practices.
- Test thoroughly: Test your application extensively to ensure compatibility with React 18 and its concurrent features.
React 18 and backward compatibility
React 18 aims to be backward compatible with previous versions, allowing developers to adopt new features gradually. Strict Mode can help you ensure compatibility by identifying potential issues during development.
Gradual adoption of React 18 features
React 18 is designed to allow gradual adoption of its features. You can start by enabling Strict Mode and fixing any issues it identifies. Next, explore concurrent rendering and other new features, adopting them in your application as needed.
Exploring other React 18 features
In addition to concurrent rendering, React 18 brings several other enhancements, such as:
- Automatic batching of updates
- Improved server-side rendering with streaming
- New suspense APIs for improved data fetching
These features unlock new capabilities and performance improvements for your React applications.
Conclusion
React Strict Mode is a valuable tool for developers upgrading to React 18 and adopting its concurrent features. By addressing issues detected by Strict Mode and following best practices, you can ensure a smooth transition to React 18 and unlock its powerful new features. Don't forget to explore other enhancements in React 18, such as automatic batching of updates, improved server-side rendering, and new suspense APIs.
FAQs
1. What is React Strict Mode?
React Strict Mode is a development tool that helps developers identify potential issues in their React applications. It doesn't affect production behavior but logs extra warnings and double-invokes functions that are expected to be idempotent during development.
2. How do I enable React Strict Mode?
To enable React Strict Mode, wrap your application or specific components in the <React.StrictMode>
component.
3. Does React Strict Mode affect production builds?
No, React Strict Mode does not affect production builds. It is only active during development and does not impact the behavior of production applications.
4. What is the purpose of React.StrictMode in the context of React 18?
React.StrictMode is essential for developers upgrading to React 18 and adopting its concurrent features. It helps identify concurrency-related bugs and other potential issues during development, ensuring a smoother transition to React 18.
5. How does React.StrictMode affect hooks like useEffect?
In Strict Mode, components will be mounted twice, which means useEffect with an empty dependency array will also be called twice. You can use useRef to manage initial actions and ensure they only run once, even when Strict Mode is enabled.