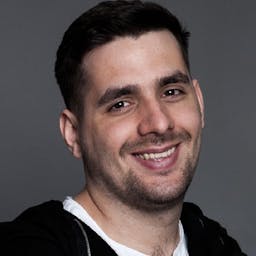
Janek Kruczkowski
Building an E-Commerce Site with React.js: A Comprehensive Case Study and Best Practices
Introduction
E-commerce has taken the world by storm, and businesses are increasingly looking for ways to build efficient and user-friendly online stores. In this article, we'll dive into a comprehensive case study on building an e-commerce site using React.js while highlighting the best practices that ensure a smooth and efficient development process.
Why React.js for e-commerce?
React.js is a popular JavaScript library for building user interfaces, created by Facebook. It offers several benefits that make it an ideal choice for e-commerce sites:
- Component-based architecture: React.js makes it easy to build reusable UI components, which can improve the maintainability and consistency of your application.
- Performance: React.js optimizes performance by minimizing unnecessary DOM updates through a virtual DOM.
- Ecosystem: A rich ecosystem of third-party libraries and tools make it easy to extend and customize your application.
Prerequisites
To follow along with this case study, you should have a basic understanding of HTML, CSS, JavaScript, and React.js.
Project setup
We'll start by setting up our project using create-react-app
, a popular tool for bootstrapping React applications. Next, we'll organize our project structure to separate components, Redux files, and assets.
Create-react-app
To create a new React project, run the following command:
npx create-react-app ecommerce-react
Project structure
Here's an example of a recommended project structure:
ecommerce-react/
├── src/
│ ├── components/
│ ├── redux/
│ │ ├── actions/
│ │ ├── reducers/
│ │ └── store.js
│ ├── assets/
│ └── App.js
└── public/
Designing the UI
UI components
For our e-commerce site, we'll need the following UI components:
- Header and navigation
- Product list and grid
- Product details page
- Shopping cart
- Checkout process
- User authentication
Material-UI
To speed up
the development process and maintain a consistent look and feel, we'll use Material-UI, a popular React UI framework based on Google's Material Design.
To install Material-UI, run the following command:
npm install @mui/material @emotion/react @emotion/styled
Setting up state management
Redux
To manage the state of our application, we'll use Redux, a popular state management library for React applications. Install Redux and its required dependencies using the following command:
npm install redux react-redux redux-thunk
Actions and reducers
We'll create actions and reducers for fetching products, handling user authentication, and managing the shopping cart.
Building product catalog
Fetching products
To display products on our e-commerce site, we'll need to fetch product data from a backend API. For this case study, we'll use a mock API, but you can replace it with any real API.
// src/redux/actions/productActions.js
import axios from 'axios';
export const fetchProducts = () => async (dispatch) => {
const response = await axios.get('https://fakestoreapi.com/products');
dispatch({ type: 'FETCH_PRODUCTS', payload: response.data });
};
Product list and grid
Using the fetched product data, we'll create a ProductList
component that displays the products in a grid layout. Each product will be represented by a ProductCard
component.
// src/components/ProductList.js
import React, { useEffect } from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { fetchProducts } from '../redux/actions/productActions';
import ProductCard from './ProductCard';
const ProductList = () => {
const products = useSelector((state) => state.products);
const dispatch = useDispatch();
useEffect(() => {
dispatch(fetchProducts());
}, [dispatch]);
return (
<div>
{products.map((product) => (
<ProductCard key={product.id} product={product} />
))}
</div>
);
};
export default ProductList;
Product details page
Routing
To enable navigation between different pages in our application, we'll use React Router, a popular routing library for React.
First, install React Router using the following command:
npm install react-router-dom
Next, set up the routes for the product details page and other pages in your App.js
file.
// src/App.js
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import ProductList from './components/ProductList';
import ProductDetails from './components/ProductDetails';
function App() {
return (
<Router>
<Switch>
<Route path="/" exact component={ProductList} />
<Route path="/product/:id" component={ProductDetails} />
</Switch>
</Router>
);
}
export default App;
Displaying product information
In the ProductDetails
component, we'll fetch and display detailed information about the selected product, such as images, description, and price.
// src/components/ProductDetails.js
import React, { useEffect } from 'react';
import { useParams } from 'react-router-dom';
import { useSelector, useDispatch } from 'react-redux';
import { fetchProductDetails } from '../redux/actions/productActions';
const ProductDetails = () => {
const { id } = useParams();
const product = useSelector((state) => state.productDetails);
const dispatch = useDispatch();
useEffect(() => {
dispatch(fetchProductDetails(id));
}, [dispatch, id]);
return (
<div>
<h2>{product.title}</h2>
<img src={product.image} alt={product.title} />
<p>{product.description}</p>
<p>Price: ${product.price}</p>
</div>
);
};
export default ProductDetails;
Shopping cart
Adding products
To allow users to add products to their shopping cart, we'll create an addToCart
action and corresponding reducer.
// src/redux/actions/cartActions.js
export const addToCart = (product) => ({
type: 'ADD_TO_CART',
payload: product,
});
In the ProductCard
component, we'll add a button that dispatches the addToCart
action when clicked.
// src/components/ProductCard.js
import React from 'react';
import { useDispatch } from 'react-redux';
import { addToCart } from '../redux/actions/cartActions';
const ProductCard = ({ product }) => {
const dispatch = useDispatch();
return (
<div>
<img src={product.image} alt={product.title} />
<h3>{product.title}</h3>
<p>Price: ${product.price}</p>
<button onClick={() => dispatch(addToCart(product))}>
Add to Cart
</button>
</div>
);
};
export default ProductCard;
Managing cart state
To display and manage the shopping cart, we'll create a Cart
component that shows the list of products in the cart, the total price, and a button to proceed to checkout.
// src/components/Cart.js
import React from 'react';
import { useSelector } from 'react-redux';
const Cart = () => {
const cartItems = useSelector((state) => state.cart);
const totalPrice = cartItems.reduce(
(total, item) => total + item.price * item.quantity,
0
);
return (
<div>
{cartItems.map((item) => (
<div key={item.id}>
<h4>{item.title}</h4>
<p>Quantity: {item.quantity}</p>
</div>
))}
<p>Total: ${totalPrice.toFixed(2)}</p>
<button>Proceed to Checkout</button>
</div>
);
};
export default Cart;
Checkout process
Forms and validation
To handle the checkout process, we'll create a Checkout
component that contains a form for the user to enter their shipping and payment information. We'll use the react-hook-form
library for form handling and validation.
First, install react-hook-form
using the following command:
npm install react-hook-form
Next, create a Checkout
component with a form for the user's information.
// src/components/Checkout.js
import React from 'react';
import { useForm } from 'react-hook-form';
const Checkout = () => {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
{/* Form fields and validation */}
<button type="submit">Place Order</button>
</form>
);
};
export default Checkout;
Handling payments
To process payments, you can integrate a third-party payment gateway, such as Stripe or PayPal. Follow their documentation to set up and configure the payment processing in your Checkout
component.
User authentication
Sign up and log in
To handle user authentication, we'll create SignUp
and LogIn
components with forms for users to enter
their email and password. We'll use the react-hook-form
library for form handling and validation.
// src/components/SignUp.js
import React from 'react';
import { useForm } from 'react-hook-form';
const SignUp = () => {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
{/* Form fields and validation */}
<button type="submit">Sign Up</button>
</form>
);
};
export default SignUp;
// src/components/LogIn.js
import React from 'react';
import { useForm } from 'react-hook-form';
const LogIn = () => {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
{/* Form fields and validation */}
<button type="submit">Log In</button>
</form>
);
};
export default LogIn;
JWT tokens
To manage user sessions, we'll use JSON Web Tokens (JWT). When a user signs up or logs in, the server will generate a JWT that is sent to the client. The client stores the token and includes it in the Authorization
header of subsequent API requests, allowing the server to verify the user's identity.
Deploying the application
Hosting options
Several hosting providers, such as Netlify, Vercel, and Firebase, offer seamless deployment and hosting options for React applications. Choose the one that best suits your needs and follow their documentation to deploy your e-commerce site.
Continuous deployment
To ensure your site stays up-to-date with the latest code changes, set up continuous deployment using your hosting provider's built-in features or external tools like GitHub Actions or CircleCI.
Optimizing for performance
Code splitting
To reduce the initial load time of your application, use code splitting to divide your code into smaller chunks that are loaded on-demand. You can achieve this using React's built-in React.lazy()
function and dynamic imports.
Lazy loading
Implement lazy loading for components that are not immediately visible or required, such as images or modals. You can use the react-lazyload
library to achieve this easily.
SEO best practices
Server-side rendering
To improve the SEO of your React application, consider using server-side rendering (SSR) with a library like Next.js. SSR generates static HTML on the server, which allows search engines to crawl and index your site more effectively.
Meta tags
Use appropriate meta tags in the public/index.html
file to provide search engines with relevant information about your site, such as title, description, and keywords.
Conclusion
In this article, we've explored a comprehensive case study on building an e-commerce site using React.js, covering everything from project setup to deployment. By following these best practices, you can build a robust, performant, and user-friendly e-commerce site.
FAQs
-
Can I use other UI frameworks instead of Material-UI?
-
Yes, you can use any UI framework or library, such as Ant Design, Bootstrap, or Tailwind CSS.
-
Can I use other state management libraries instead of Redux?
-
Yes, you can use alternatives like MobX or React's built-in Context API.
-
How can I handle internationalization in my React application?
-
You can use a library like
react-i18next
to handle internationalization and localization. -
How can I handle SEO for my React application if I don't want to use server-side rendering (SSR)?
-
You can use a technique called prerendering to generate static HTML pages during the build process. Prerendering is less flexible than SSR but is easier to set up and can improve the SEO of your application. You can use tools like
react-snap
orprerender.io
to implement prerendering. -
How can I optimize the performance of my React application?
-
In addition to code splitting and lazy loading, you can optimize your application's performance by using React's built-in performance profiling tools, implementing memoization with
React.memo
, and optimizing the rendering process withReact.PureComponent
orshouldComponentUpdate
.